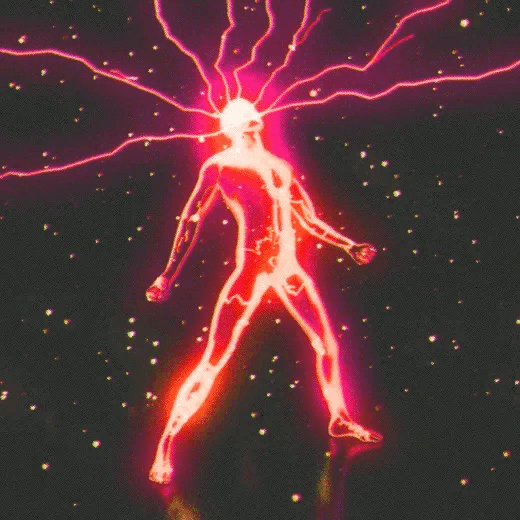
Into the Future with Glitch
My first experience building a Glitch web app. I used the default Glitch setup of Node.js with Express on some fascinating historical data freely available from an open-source project.
Why Glitch?
Glitch made me feel like I was living in the future. As I was building a Node app on Glitch, I found it amusing to come across Node tutorials that said things like "make sure Node is installed on your computer". After just one day of using Glitch this advice feels as archaic as a floppy disk. As a Glitch developer, I don't concern myself with hardware. I don't install things on my computer. Suddenly the idea of a local development environment seems quaint.
This blog post describes my first Glitch project, Love & Seduction in the 19th Century. It's a very simple app that displays randomly-selected text when a user pushes a button. The text source is data from a digital humanities project.
Getting started with Glitch is so simple that it's almost confusing. You visit Glitch.com, press the button that says New Project, and select one of three project types. At that point you have a running, deployed project. Everything else is (or can be) just a matter of customizing this default project.
Here's how the button looks:
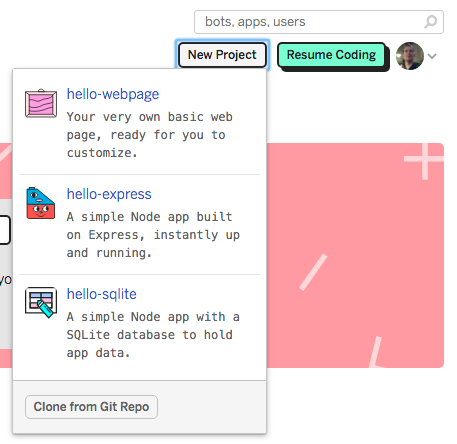
Client-side code
The default Glitch Express Node project comes with a public/client.js file containing all the code that makes the default project work. This is client-side code, executed by the visiting user's web browser. After my modifications, my client-side JavaScript looks like this:
// client-side js// run by the browser each time your view template is loaded
// a function to connect to server and get text
const findLove = async function() {
let love = await axios.get('/text');
return love['data']; };
// define variables that reference elements on our page
const loveForm = document.forms[0];
const loveBox = document.getElementById('lovebox');
// a helper function that adds the text into the HTML
const showLove = function(love) {
loveBox.innerHTML = love; }
// listen for button to be pressed, replace the text
loveForm.onsubmit = async function(event) {
// stop form submission from refreshing the page
event.preventDefault();
// select a random text item
love = await findLove();
showLove(love); };
I've repurposed and renamed code that interacts with the HTML form in the views/index.html page. (I haven't included my modifications to the index page in this blog post. But, because every Glitch app is open source by default, you can check them out for yourself!)
The first bit of my public/client.js file contains an HTTPS connection to a path within the project. This connection is the channel through which my client-side JavaScript shall connect with server-side code. The server will provide the client with information that it will use to update what the user sees in their browser.
This is the code for the connection to the data source:
// a function to connect to server and get textconst findLove = async function() {
let love = await axios.get('/text');
return love['data']; };
Thanks to Cassey Lottman for the advice to use the Axios library as an alternative to the default XMLHttpRequest technique. Thanks to her, also, for suggesting the await operator to tell my client-side JavaScript to wait for a response from the server before proceeding. This helped me deal with mysteries of asynchronous code!
Server-side code
My server-side code, which lives in the server.js file, connects to a source of data and it provides an API method through which a randomly-chosen item from that data is transmitted:
// server.js// where your node app starts
// init project
const express = require('express');
const app = express();
// Express routes included in default project
app.use(express.static('public'));
app.get('/', function(request, response) {
response.sendFile(__dirname + '/views/index.html'); });
// create array of data from file
const loves = require('./data.json');
// a route so client can connect to get data
app.get('/text', function(request, response) {
response.send(loves['text'][Math.floor(Math.random()*loves['text'].length )]); });
// listen for requests
const listener = app.listen(process.env.PORT, function() {
console.log('Your app is listening on port ' + listener.address().port); });
My data are text excerpts from literature, court cases, journals and other documents dating from the 19th Century. The documents are part of a project of the University of Nebraska, hosted by the Center for Digital Research in the Humanities. The project archives historical materials on the history of love and seduction. It is open source. Thanks to Jessica Dussault for pointing me to this fascinating, free material!
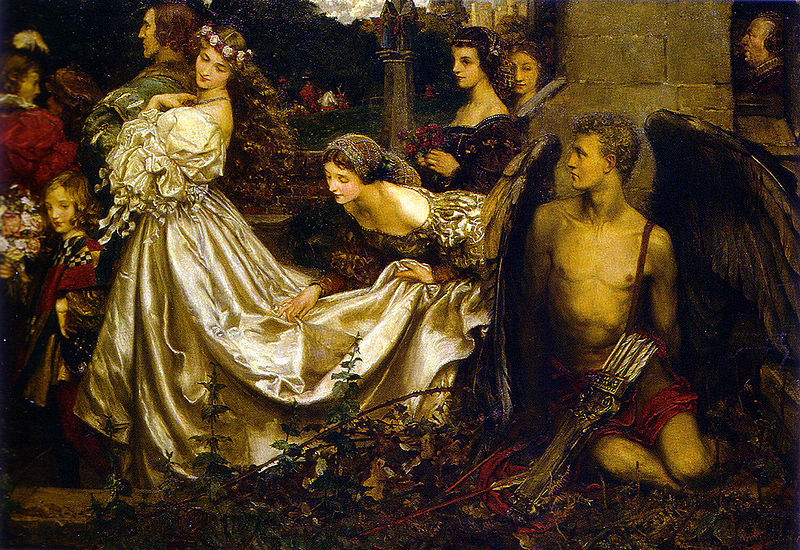
Note: the 19th Century was horrible. I've selected text snippets for my project that seem like fun in isolation. If you read many of them in context you'll be reminded what extraordinary misogyny and stultifying social conventions were widespread in uncomfortably recent times.
These strings are stored in a .json file that I'm treating as if it were a database. My server-side JS requires the data file to get access to it, then chooses a random string with:
loves['text'][Math.floor( Math.random()*loves['text'].length )];I find that code for random selection of an item from an array to be long and clumsy, but I guess that's how it's done in JS.
The finished Glitch web app
All of this functionality could have been built entirely as front-end code. But, I like the idea of having the data access and selection take place on a server. In the future I may decide to store data in another way, such as in a real database. My choice to locate data logic on the server means I'll not need to change client-side code if my data storage method changes.
Now that I've written the app, it is deployed. Everything we create on glitch snaps to attention immediately, keystroke for keystroke.
The project detailed in this blog post is running here and I've also embedded it below. Have fun!